[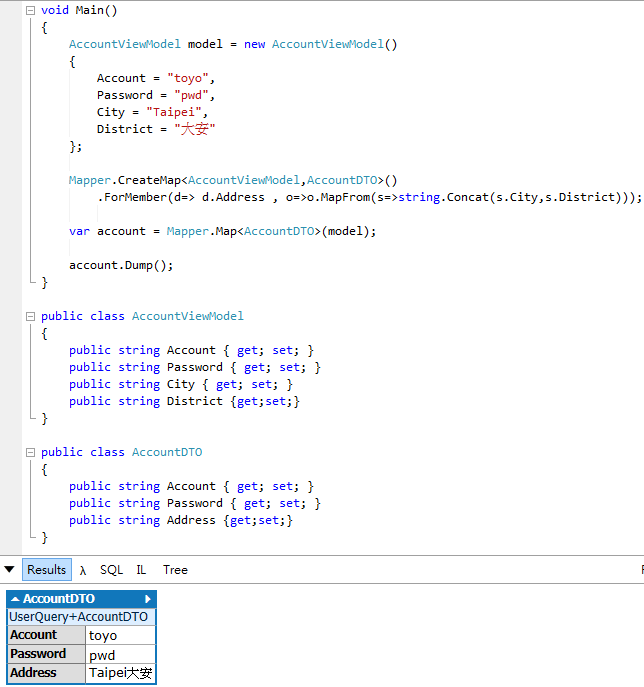](http://1.bp.blogspot.com/-n04XweFHx5w/VimuRLyJtGI/AAAAAAAAHgg/H0pNXYnW11w/s1600/1.png) * 如果兩的類別對應到同一個物件的情況 ```csharp void Main() { AccountViewModel model = new AccountViewModel() { Account = "toyo", Password = "pwd", City = "Taipei", District = "大安" }; var Group = new Group() { ID = 1, Name = "客戶群組" }; Mapper.CreateMap<AccountViewModel,AccountDTO>() .ForMember(d=> d.Address , o=>o.MapFrom(s=>string.Concat(s.City,s.District))); var account = Mapper.Map<AccountDTO>(model);
//這邊用上面已經轉好的account,來接續Mapper Mapper.CreateMap<Group, AccountDTO>() .ForMember(d => d.Account, o => o.Ignore()) .ForMember(d => d.Password, o => o.Ignore()) .ForMember(d => d.Address, o => o.Ignore()) .ForMember(d => d.GroupName, o => o.MapFrom(s=>s.Name)); //注意 account = Mapper.Map<Group,AccountDTO>(Group,account);
account.Dump(); }
public class AccountViewModel { public string Account { get; set; } public string Password { get; set; } public string City { get; set; } public string District {get;set;} }
public class AccountDTO { public string Account { get; set; } public string Password { get; set; } public string Address { get; set; } public string GroupName {get;set;} }
public class Group{ public int ID { get; set; } public string Name {get;set;} }